Hey there, Golang enthusiasts! If you're diving into the world of Go programming, one of the things you'll inevitably encounter is figuring out how to check the type of a variable. This guide will walk you through everything you need to know about checking variable types in Golang, from the basics to advanced techniques. Whether you're a beginner or a seasoned developer, this article has got your back.
Let's face it—working with variables in Golang can sometimes feel like solving a puzzle, especially when you're dealing with interfaces and dynamic data. But don't worry; we'll break it down step by step so you can confidently check the type of any variable without breaking a sweat.
By the time you finish reading this, you'll have a solid understanding of how to work with Go's type system, use type assertions, and even implement type switches. Ready to level up your Golang game? Let's dive in!
Read also:Top Male Actors Under 20 Rising Stars Shaping Hollywoods Future
Table of Contents
- Introduction to Golang Variable Types
- Why You Should Check Variable Types
- Basic Methods to Check Variable Types
- Using Type Assertions
- Implementing Type Switches
- Working with Interfaces
- Common Mistakes to Avoid
- Best Practices for Type Checking
- Real-World Examples
- Wrapping It All Up
Introduction to Golang Variable Types
Alright, let's start with the basics. In Golang, variables are like containers that hold data. But here's the twist—Go is statically typed, which means the type of a variable is determined at compile time. So, why does checking variable types matter? Well, sometimes you'll deal with interfaces or receive data from external sources, and you'll need to know what you're working with before proceeding.
For example, imagine you're building an API that accepts JSON data. The incoming data might be a string, integer, or even a nested object. How do you ensure your program behaves correctly? That's where type checking comes in.
Now, let's explore why this is crucial in Golang development.
Why You Should Check Variable Types
Checking variable types in Golang isn't just about curiosity—it's about ensuring your code behaves as expected. Here are a few reasons why this practice is essential:
- Prevent Errors: By verifying types, you reduce the risk of runtime errors caused by unexpected data.
- Enhance Code Reliability: Type checking adds an extra layer of safety, making your code more robust.
- Improve Readability: Clear type handling makes your code easier to understand for yourself and others.
- Work with Interfaces: When dealing with interfaces, type checking is often necessary to access the underlying data.
So, whether you're building small scripts or large-scale applications, mastering type checking will take your Golang skills to the next level.
Basic Methods to Check Variable Types
Now that we've covered why type checking matters, let's dive into the practical side. There are several ways to check variable types in Golang. Here's a quick rundown:
Read also:How Tall Is Melli Monaco Unlocking The Secrets Behind This Rising Star
Using the type
Keyword
Go provides a straightforward way to declare and check types using the type
keyword. For instance:
var myVar int
In this case, myVar
is explicitly declared as an integer. While this doesn't directly check the type, it ensures the variable adheres to the specified type.
Using Reflection
Golang's reflect
package is your best friend when it comes to inspecting variable types dynamically. Here's an example:
import "reflect"
myVar := 42
fmt.Println(reflect.TypeOf(myVar))
This will output int
, indicating the type of myVar
. Reflection is powerful but should be used sparingly due to potential performance impacts.
Using Type Assertions
Type assertions are another powerful tool in Golang for checking variable types, especially when working with interfaces. Here's how it works:
Single Value Assertion
var myInterface interface{} ="Hello, Golang!"
str, ok := myInterface.(string)
if ok {
fmt.Println("It's a string:", str)
}
In this example, we check if myInterface
holds a string. If it does, the assertion succeeds, and str
holds the value.
Multiple Value Assertion
myVar, ok := myInterface.(int)
if !ok {
fmt.Println("Not an integer")
}
This approach allows you to handle cases where the type doesn't match gracefully.
Implementing Type Switches
When dealing with multiple possible types, type switches come in handy. They're similar to regular switch statements but tailored for type checking. Here's an example:
switch v := myInterface.(type) {
case int:
fmt.Println("Integer:", v)
case string:
fmt.Println("String:", v)
default:
fmt.Println("Unknown type")
}
This code snippet checks the type of myInterface
and executes the corresponding block based on the result.
Working with Interfaces
Interfaces in Golang are a powerful feature that allows you to define behavior without specifying the underlying type. However, when you need to access the actual type, type checking becomes essential.
Embedding Interfaces
type MyInterface interface {
Method1()
}
func checkType(i MyInterface) {
switch v := i.(type) {
case *ConcreteType:
fmt.Println("ConcreteType detected:", v)
default:
fmt.Println("Unknown type")
}
}
This example demonstrates how to embed interfaces and check the underlying type.
Common Mistakes to Avoid
Even the best developers make mistakes, and type checking in Golang is no exception. Here are some pitfalls to watch out for:
- Overusing Reflection: While reflection is powerful, it can slow down your application if used excessively.
- Ignoring Errors: Always handle errors from type assertions to avoid unexpected behavior.
- Not Documenting Code: Clearly document your type checks to help future developers understand your logic.
By avoiding these common mistakes, you'll write cleaner, more efficient Golang code.
Best Practices for Type Checking
Now that you know the dos and don'ts, here are some best practices to keep in mind:
- Keep It Simple: Use type assertions and switches only when necessary; rely on Go's static typing whenever possible.
- Optimize Performance: Avoid unnecessary type checks that could impact your application's speed.
- Write Tests: Test your type-checking logic thoroughly to ensure it works as expected in all scenarios.
Following these practices will help you write maintainable, efficient Golang code.
Real-World Examples
Let's look at a couple of real-world examples where type checking in Golang proves invaluable:
Handling JSON Data
When working with JSON, you often receive data as interfaces. Here's how you might handle it:
data := map[string]interface{}{"name": "John", "age": 30}
name, ok := data["name"].(string)
if ok {
fmt.Println("Name:", name)
}
This example extracts the name from a JSON-like structure, ensuring it's a string.
Building APIs
In API development, type checking ensures incoming data matches expected formats:
type User struct {
Name string
}
func createUser(user interface{}) {
u, ok := user.(User)
if !ok {
fmt.Println("Invalid user data")
return
}
fmt.Println("Creating user:", u.Name)
}
This snippet demonstrates how to validate incoming user data before processing it.
Wrapping It All Up
And there you have it—a comprehensive guide to checking variable types in Golang. From basic methods to advanced techniques, you now have the tools to confidently handle any type-related challenges in your Golang projects.
Remember, mastering type checking isn't just about writing code—it's about writing safe, reliable, and efficient code. So, keep practicing, stay curious, and don't forget to share your newfound knowledge with fellow developers!
Got any questions or tips of your own? Drop a comment below and let's keep the conversation going. Happy coding, and see you in the next one!
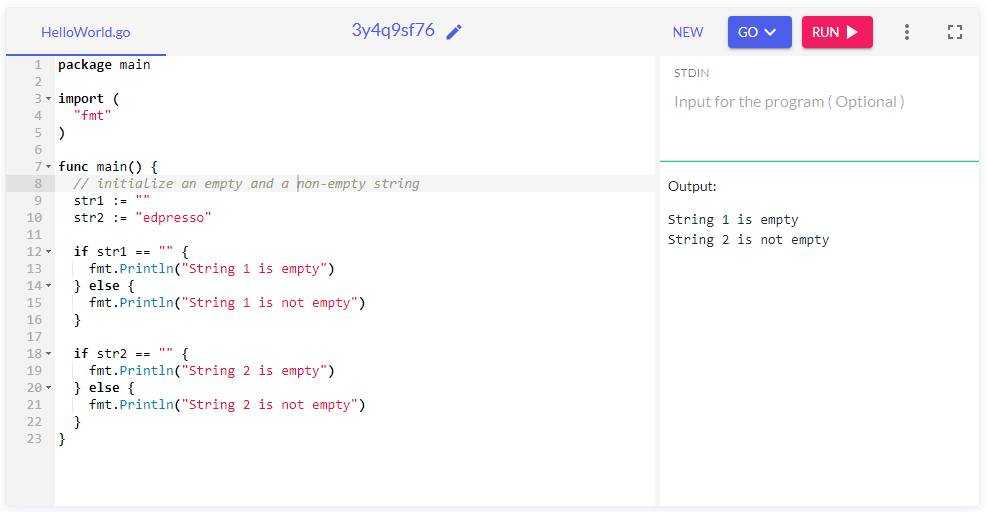
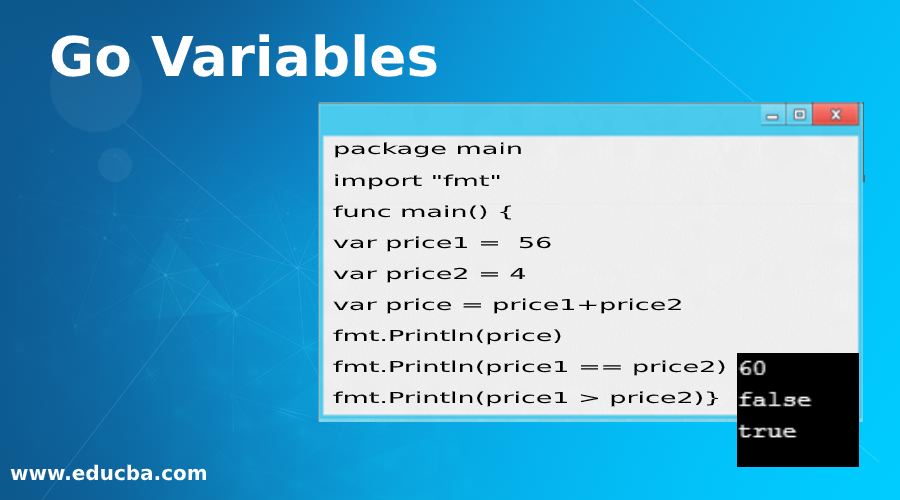
